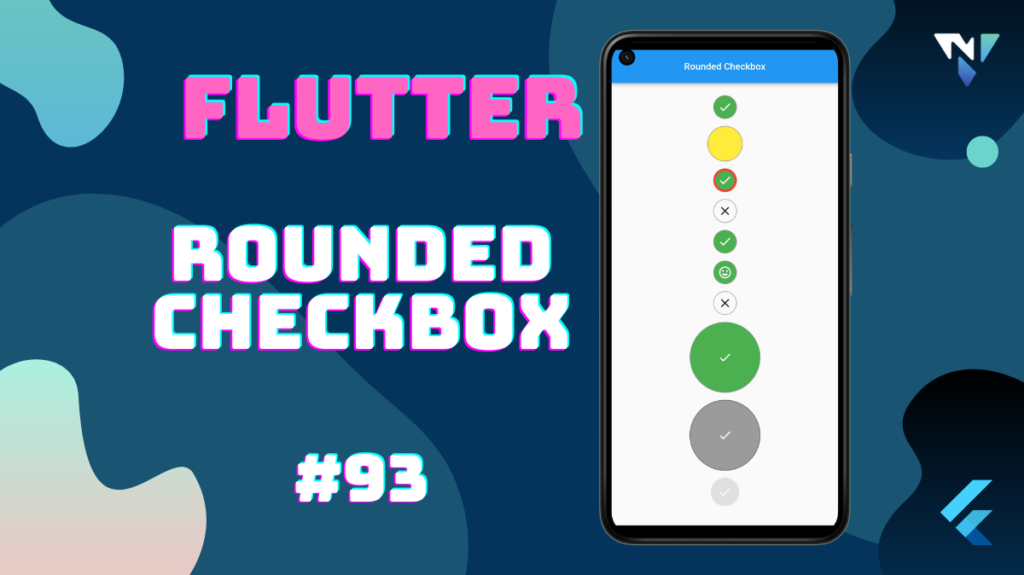
Tutorial and code of Rounded Checkbox in Flutter. Copy and paste the below code as per your requirements.
roundcheckbox: ^2.0.4+1
import 'package:flutter/material.dart';
import 'package:roundcheckbox/roundcheckbox.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: const CheckBox(),
);
}
}
class CheckBox extends StatelessWidget {
const CheckBox({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Center(child: Text("Rounded Checkbox"),),),
body: SizedBox(
width: double.infinity,
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: <Widget>[
RoundCheckBox(
onTap: (selected) {},
),
const SizedBox(height: 12),
RoundCheckBox(
onTap: (selected) {},
size: 60,
uncheckedColor: Colors.yellow,
),
const SizedBox(height: 12),
RoundCheckBox(
onTap: (selected) {},
border: Border.all(
width: 4,
color: Colors.red,
),
uncheckedColor: Colors.red,
uncheckedWidget: const Icon(Icons.close, color: Colors.white),
),
const SizedBox(height: 12),
RoundCheckBox(
onTap: (selected) {},
uncheckedWidget: const Icon(Icons.close),
),
const SizedBox(height: 12),
RoundCheckBox(
onTap: (selected) {},
uncheckedWidget: const Icon(Icons.close),
animationDuration: const Duration(
milliseconds: 50,
),
),
const SizedBox(height: 12),
RoundCheckBox(
onTap: (selected) {},
checkedWidget: const Icon(Icons.mood, color: Colors.white),
uncheckedWidget: const Icon(Icons.mood_bad),
animationDuration: const Duration(
seconds: 1,
),
),
const SizedBox(height: 12),
RoundCheckBox(
onTap: (selected) {},
uncheckedWidget: const Icon(Icons.close),
isChecked: true,
),
const SizedBox(height: 12),
RoundCheckBox(
onTap: (selected) => print(selected),
uncheckedWidget: const Icon(Icons.close),
isChecked: true,
size: 120,
),
const SizedBox(height: 12),
const RoundCheckBox(
onTap: null,
uncheckedWidget: Icon(Icons.close),
isChecked: true,
size: 120,
),
const SizedBox(height: 12),
RoundCheckBox(
onTap: null,
uncheckedWidget: const Icon(Icons.close),
disabledColor: Colors.grey[300],
isChecked: true,
size: 48,
),
const SizedBox(height: 12),
],
),
),
);
}
}